Part 2-API testing with rest assured.
- khyati sehgal
- Sep 20, 2014
- 2 min read
In continuation with my previous blog, here I will be sharing some basic rest services methods implementation like POST, GET etc.
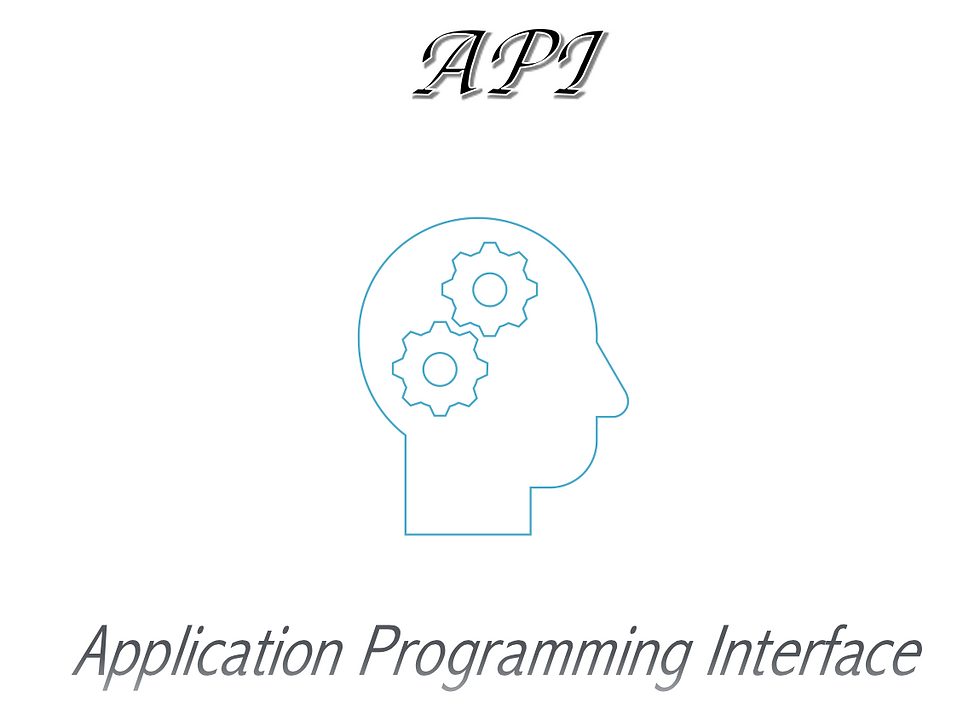
REST in itself without exception has a lot to learn. It has helped the whole team of developers and QA to evolve and mobilise their work as much as possible.
By providing whole lot of the methods like GET, POST, PUT, DELETE, PATCH, etc one can traverse the whole code. Not just fetching, or updating, one can even delete then store and use one data in one and another form. Thus making a complete round of testing and development done.
Like REST java also helped in making this dynamic thing more super, lets see how.
How to create a basic POST function in rest assured with java?
Before starting with the actual code there are some keywords which you shall be familiar with to understand the code fully.
Rest follow a protocol while writing code, it follows it in a user story way or Behaviour driven way aka BDD which is given, when and then. I am capturing the whole response in a static variable. And then using the value of that variable I am verifying and playing with the different information that can be extracted from it.
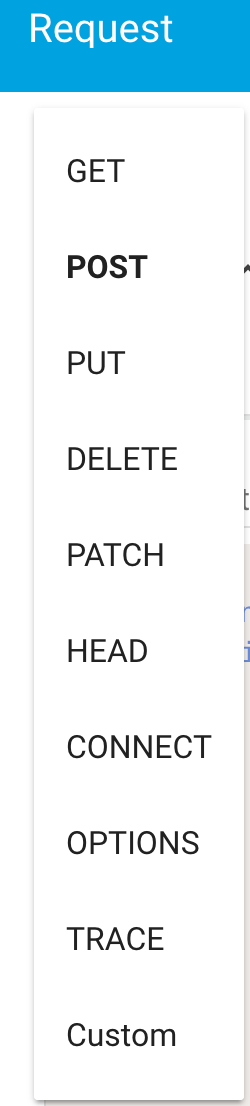
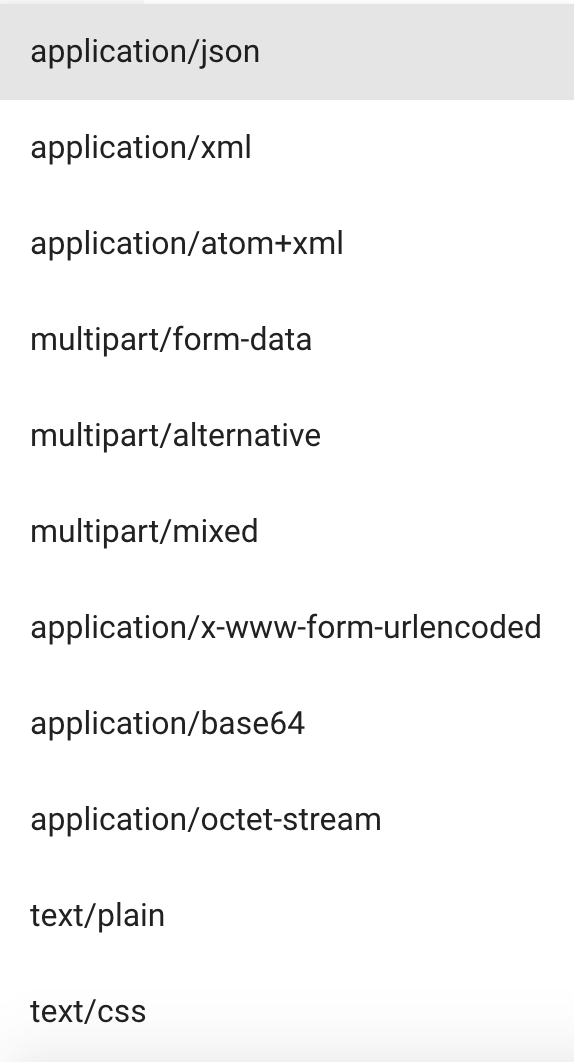
given is a pre condition which shall be provided to the service before calling it.
header can be used for Setting a header like application/json , application/xml, form-data, etc.
post is a method of rest service , can vary according to the requirement.
then is a post condition which shall be seen once the API is hit.
statusCode set if status which HTTP/HTTPs provides like 200, 400, 400, 500, 302, etc. for each status code a status message is mapped in rest assured like SC_OK for 200OK, SC_BAD_REQUEST for 400, etc.
contentType is the expected content type which should come once the service will be called.
Then depending on the requirement assertions can be called like verifying auth_token, status code, time taken etc.
NOTE: I have created a generic method called fieldValues which I will be calling in every method as a general verification.
// Post API to Login as Admin and get Authorization Token
public static String postRequestToAuthLogin() {
//Setting header content type as application/json
Header header = new Header("Content-Type", "application/json");
protected static Response response = given().log().all().header(header).when().body(property.getProperty("auth_token_payload"))
.post(property.getProperty("auth_login_path"))
.then().statusCode(HttpStatus.SC_OK)
.contentType(ContentType.JSON).extract().response();
String auth_token = response.path("token").toString();
Loggers.info("authentication_token is " + auth_token);
property.setProperty("auth_token", auth_token);
fieldValues();
return auth_token;
}
How to create a basic GET function in rest assured with java?
public static void getRequestToLogout() {
response = given().header("Content-Type", "application/json")
.header("Authorization", "Token " + property.getProperty("auth_token"))
.when()
.get(property.getProperty("auth_logout"))
.then().statusCode(HttpStatus.SC_OK)
.extract()
.response();
fieldValues();
}
fieldValues function
public static void fieldValues() {
statusLine = response.getStatusLine();
Loggers.info("Status line is " + statusLine);
statusCode = response.getStatusCode();
Loggers.info("Status Code is " + statusCode);
timeInMs = response.time();
Loggers.info("Time taken is " + timeInMs);
body = response.getBody().asString();
Loggers.info("Response body is" + body);
}
Depending on the verification we can put assertions on different field values we get from the response. As you have noticed I have put loggers to trace log of the output this is an optional implementation and can be modified as per the project set up.
コメント